Streamlit + FastAPI:A New Approach for Rapidly Building and Deploying AI Web Applications
- Authors
In the field of application development, especially within data science and AI applications, selecting the right tools for creating, deploying, and managing applications is crucial. This article explores a new approach to rapidly building and deploying AI web applications using Streamlit and FastAPI, detailing their architecture, working mechanisms, advantages, and practical use cases, along with providing real-world example programs.
1. Overview
Streamlit
Streamlit is an open-source Python library designed to help developers effortlessly create web applications for data projects with minimal effort. It emphasizes simplicity, allowing developers to transform data scripts into shareable web applications using concise and understandable code.
FastAPI
FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. It is intended to create robust and efficient APIs, particularly well-suited for data applications due to its quick execution and efficient data handling capabilities.
2. Streamlit + FastAPI
Streamlit as the Frontend
- Developing User Interfaces: Streamlit is used to develop the application’s user interface, providing users with an interactive and visually appealing way to interact with data.
- Data Visualization: It allows developers to create various data visualizations, such as charts and graphs, to present data in an easily understandable format.
- User Interaction: Streamlit applications can receive user input and display visual results through various Streamlit components.
FastAPI as the Backend
- Handling Business Logic: FastAPI manages the application’s business logic, processes data, executes algorithms, and ensures the application operates as intended.
- Interacting with Databases: It handles database interactions, ensuring data is stored, retrieved, updated, and deleted correctly according to the application's needs.
- Managing Data Processing Tasks: FastAPI can manage various data processing tasks, ensuring that data is correctly handled and manipulated before being sent to the frontend.
Overall Interaction Workflow
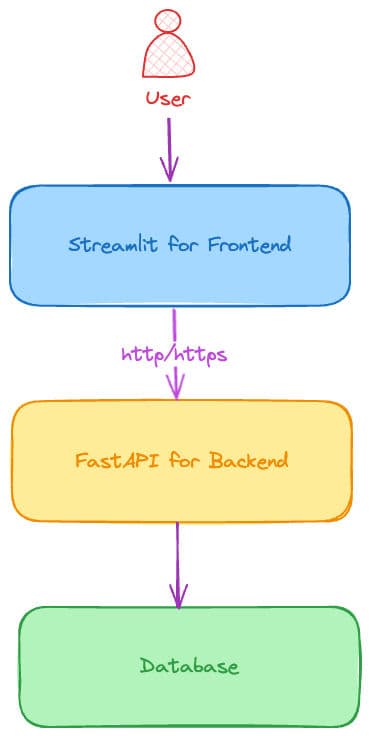
- User Interaction: Users interact with the Streamlit application, using widgets to input data and request specific visualizations or analyses.
- Data Visualization: Streamlit provides visualizations based on user input and possibly pre-processed data.
- Request Handling: When more complex data processing or retrieval is needed, Streamlit communicates with the FastAPI backend, sending requests to specific APIs.
- Data Processing: FastAPI processes the data, interacts with databases, and executes business logic as needed.
- Response: FastAPI returns the processed data to the Streamlit frontend.
- Displaying Results: Streamlit updates the displayed data and visualizations based on the processed data received from FastAPI.
This architecture allows developers to leverage Streamlit’s simplicity and interactivity for user interfaces and data visualization, while utilizing FastAPI’s robustness and efficiency for backend processing, data management, and business logic.
3. Why Use Streamlit + FastAPI Architecture
1. Rapid MVP Development
- Simplified Development: Streamlit allows developers to quickly convert data scripts into web applications without requiring deep knowledge of frontend technologies.
- Quick Iteration: Developers can immediately see the impact of code changes on the application, facilitating rapid iteration.
2. Scalability
- Asynchronous Capabilities: FastAPI supports asynchronous request handling, allowing it to manage multiple requests concurrently, which is critical for high-traffic applications.
- Performance: Known for its high performance, FastAPI ensures that applications can scale to handle increased loads without significant degradation in response time.
- Ease of Migration: If the MVP version performs well, Streamlit can be quickly replaced with a professional frontend application, while the backend FastAPI requires little to no modification.
3. Ease of Use
- Python-Based: Both Streamlit and FastAPI are Python-based, making them accessible to developers familiar with Python and ensuring a smooth learning curve.
- Simplified Syntax: The syntax of both frameworks is designed to be simple and intuitive, allowing developers to focus on building functionality rather than grappling with complex syntax.
4. Strong Community and Ecosystem
- Active Community: Both Streamlit and FastAPI have active, supportive communities that offer a wealth of knowledge, resources, and help through forums, social media, and other platforms.
- Rich Ecosystem: The ecosystems of these tools include a variety of plugins, extensions, and integrations, making it easy for developers to extend functionality and integrate with other tools and technologies.
4. Practical Example: Stock Price Visualization Application
FastAPI + Streamlit presents a new approach to creating AI applications, harnessing the strengths of both frameworks. Below is a comprehensive code example demonstrating how to integrate FastAPI with Streamlit and rapidly build and deploy an application.
FastAPI Code
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class StockRequest(BaseModel):
symbol: str
@app.post("/get_stock")
async def get_stock(data: StockRequest):
# Here you might fetch real stock data, for simplicity returning a static response
return {"symbol": data.symbol, "price": 100.0}
Streamlit Code
import streamlit as st
import requests
st.title('Stock Price App')
symbol = st.text_input("Enter Stock Symbol:", value="AAPL")
if st.button('Get Price'):
response = requests.post("http://localhost:8000/get_stock", json={"symbol": symbol})
if response.status_code == 200:
stock_data = response.json()
st.write(f"The price of {stock_data['symbol']} is ${stock_data['price']}")
else:
st.write("Error fetching the stock price")
With just a few lines of code, you can achieve the following:
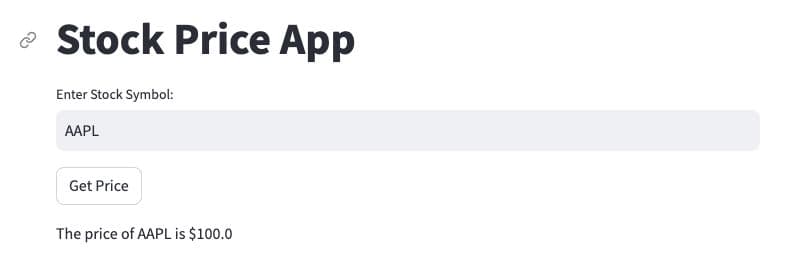
For more detailed code and building steps, you can check out my GitHub repository.
5. Conclusion
The integration of Streamlit and FastAPI offers a new approach for developing data/AI-driven web applications, combining Streamlit's simplicity with FastAPI's high performance and scalability. This not only facilitates rapid development but also ensures that applications are scalable and maintainable, with the ability to be seamlessly deployed using modern technologies and platforms.
Share this content